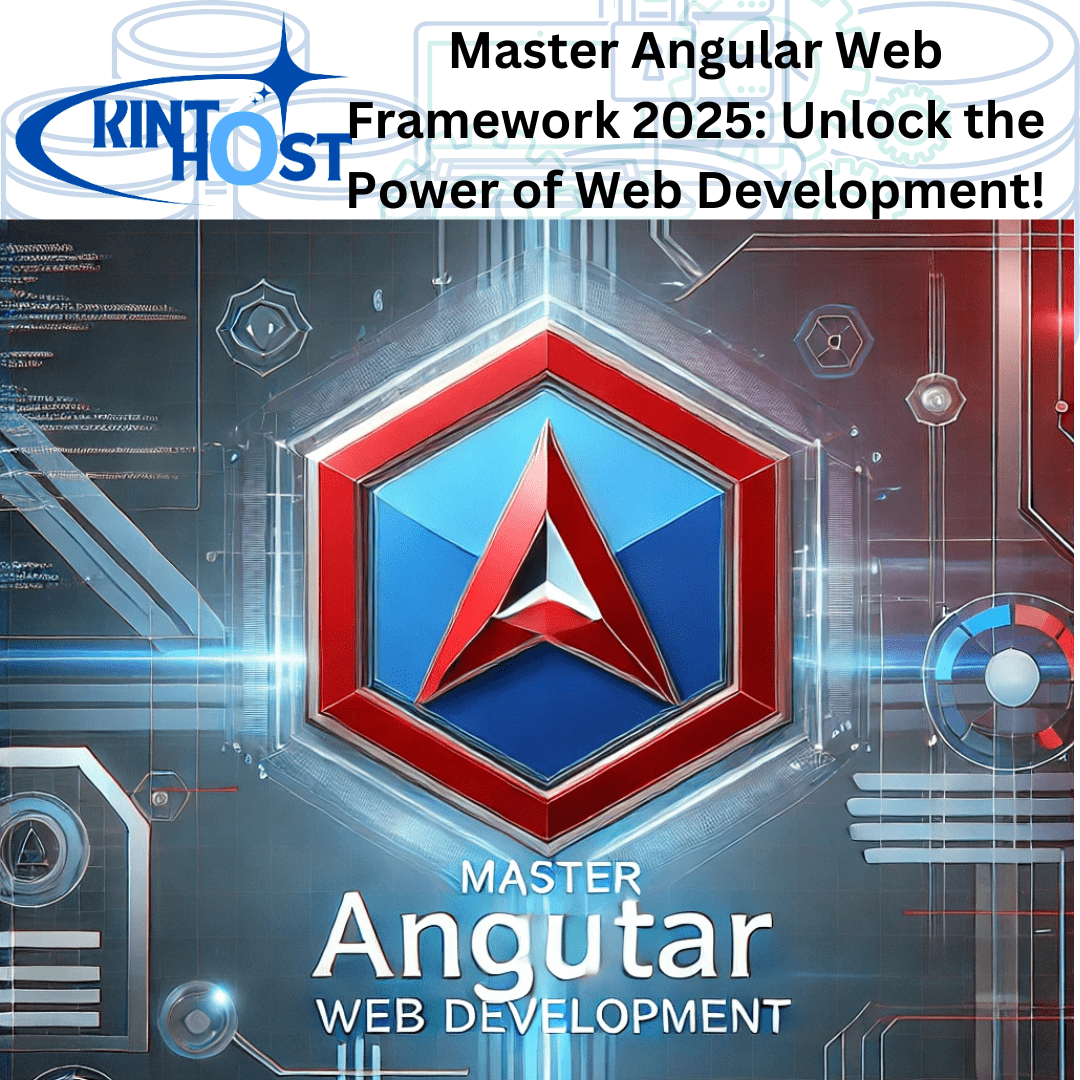
Angular Web Framework is one of the most powerful and widely used web frameworks for building dynamic, scalable, and maintainable web applications. Developed and maintained by Google, Angular Web Framework provides developers with a robust ecosystem for creating feature-rich applications using TypeScript. In this comprehensive guide, we will explore everything you need to know about Angular, from its architecture and key features to best practices and SEO optimization techniques.
What is Angular Web Framework?
Angular Web Framework is an open-source, front-end framework based on TypeScript and designed to build Single Page Applications (SPAs). It follows the component-based architecture, making development modular and maintainable. Unlike traditional JavaScript frameworks, Angular offers built-in solutions for dependency injection, state management, and routing, eliminating the need for additional third-party libraries.
Key Features of Angular Web Framework
- Component-Based Architecture
- Angular Web Framework applications are structured using components, making code reusable and easier to manage.
- Two-Way Data Binding
- Angular Web Framework synchronizes the model and the view, reducing boilerplate code.
- Dependency Injection (DI)
- Built-in DI makes it easy to manage dependencies and improve testability.
- Directives and Templates
- Custom directives extend HTML functionalities, while templates define the UI.
- Routing and Navigation
- The Angular Router enables seamless navigation between views.
- Built-in Form Handling
- Supports both reactive and template-driven forms.
- Performance Optimization
- Lazy loading, Ahead-of-Time (AOT) compilation, and Change Detection strategies enhance performance.
- SEO-Friendly
- Server-Side Rendering (SSR) with Angular Universal improves search engine visibility.
- Comprehensive Testing Support
- Jasmine and Karma for unit and end-to-end testing.
- Cross-Platform Compatibility
- Build progressive web apps (PWAs) and mobile applications with Angular.
Why Choose Angular for Web Development?
- Backed by Google
- Regular updates, strong community support, and a robust ecosystem.
- Enterprise-Grade Applications
- Suitable for large-scale applications requiring high maintainability.
- TypeScript Advantage
- Ensures better tooling, code consistency, and enhanced error handling.
- Scalability and Maintainability
- Modular architecture allows easy scaling and management of code.
Angular Architecture Explained
1. Modules
Angular Web Framework applications are modular and divided into NgModules. Each module consolidates components, directives, pipes, and services.
2. Components
The UI of an Angular application is built using components, which consist of:
- Template: Defines the HTML structure.
- Class: Contains the business logic written in TypeScript.
- Styles: CSS or SCSS files to define component-specific styles.
3. Templates and Directives
Angular templates use directives such as *ngFor
and *ngIf
to manipulate the DOM dynamically.
4. Services and Dependency Injection
Services are used to share data and logic between components. The Dependency Injection system ensures efficient management of service instances.
5. Routing and Navigation
The Angular Router module manages navigation between different application views.
6. State Management
For complex applications, state management libraries like NgRx and Akita help maintain application state efficiently.
Getting Started with Angular
1. Installing Angular CLI
npm install -g @angular/cli
2. Creating a New Angular Project
ng new my-angular-app
cd my-angular-app
ng serve
3. Project Structure
The Angular project contains several directories, including src
, app
, assets
, and environments
.
4. Creating Components
ng generate component my-component
Best Practices for Angular Development
- Use Lazy Loading
- Reduces initial load time by loading modules only when needed.
- Optimize Change Detection
- Use
OnPush
strategy to improve performance.
- Use
- Implement State Management
- Use NgRx or BehaviorSubject for efficient state management.
- Follow Folder Structure Best Practices
- Organize modules, services, and components logically.
- Use Environment Variables
- Manage different API configurations for development and production environments.
SEO Optimization in Angular
Since Angular is a SPA framework, SEO optimization requires additional techniques to ensure proper indexing.
1. Use Angular Universal for SSR
Angular Universal enables Server-Side Rendering (SSR), improving search engine visibility.
ng add @nguniversal/express-engine
2. Meta Tags and Titles
Dynamically set meta tags for better SEO using Angular’s Meta service.
import { Meta, Title } from '@angular/platform-browser';
constructor(private meta: Meta, private title: Title) {
this.title.setTitle('Best Angular Web Development Guide');
this.meta.updateTag({ name: 'description', content: 'Learn Angular with this comprehensive guide.' });
}
3. Optimize Page Load Speed
- Implement lazy loading and AOT compilation.
- Use efficient image formats and minify CSS/JS files.
4. Structured Data Markup
Use JSON-LD for rich snippets to enhance search engine ranking.
Angular Hosting and Deployment
1. Choosing the Right Hosting Provider
To ensure seamless deployment, consider KintoHost for high-performance Angular hosting.
2. Deploying to Firebase
ng add @angular/fire
ng deploy
3. Using Docker for Deployment
Dockerizing Angular applications enhances portability and scalability.
Real-World Applications of Angular
1. E-commerce Websites
Angular is widely used in building scalable e-commerce platforms. For example, Indian Shop Hub offers seamless shopping experiences using modern web technologies.
2. Food Delivery Applications
Platforms like Uniq Foody leverage Angular to create smooth, interactive user interfaces for food delivery services.
3. Perfume E-commerce Websites
Modern perfume websites like VVID Perfumes use Angular’s component-based structure to enhance UI/UX.
4. Auto Refresh Applications
Applications like Auto Refresh use Angular’s real-time data updates and reactive programming capabilities.
Conclusion
Angular Web Framework is a robust framework for building scalable and maintainable web applications. With features like component-based architecture, two-way data binding, and dependency injection, it offers unparalleled advantages to developers. By following best practices, optimizing for SEO, and choosing reliable hosting, businesses can maximize the potential of Angular for their web applications.
10 Frequently Asked Questions (FAQs) About Angular
- What is Angular mainly used for?
- Angular is used for building dynamic, single-page web applications (SPAs) with a structured and modular approach.
- How does Angular differ from React and Vue?
- Angular Web Framework is a full-fledged framework with built-in features like dependency injection and routing, whereas React and Vue are more lightweight and component-based libraries.
- What are the benefits of using TypeScript in Angular?
- TypeScript provides static typing, better tooling, and improved code maintainability, making Angular applications more scalable.
- How can I optimize an Angular application for SEO?
- Using Angular Universal for server-side rendering (SSR), setting meta tags dynamically, and optimizing page load speed with lazy loading.
- What is the best state management solution for Angular?
- Angular Web Framework offers built-in services, but third-party libraries like NgRx, Akita, and BehaviorSubject are popular choices for managing application state.
- How do I deploy an Angular app?
- You can deploy an Angular Web Framework app using hosting services like Firebase, Netlify, Vercel, or custom cloud hosting such as AWS and KintoHost.
- What are some common challenges faced in Angular development?
- Performance optimization, SEO challenges in SPAs, managing state in large applications, and handling asynchronous operations efficiently.
- How does Angular handle form validation?
- Angular Web Framework provides both template-driven and reactive forms, offering built-in validation techniques like required fields, custom validators, and async validation.
- What is lazy loading in Angular?
- Lazy loading helps improve performance by loading modules only when they are needed, reducing the initial bundle size.
- How do I secure an Angular application?
- Implement authentication using JWT, use Angular’s built-in security features like sanitization, and enable HTTPS and Content Security Policy (CSP).