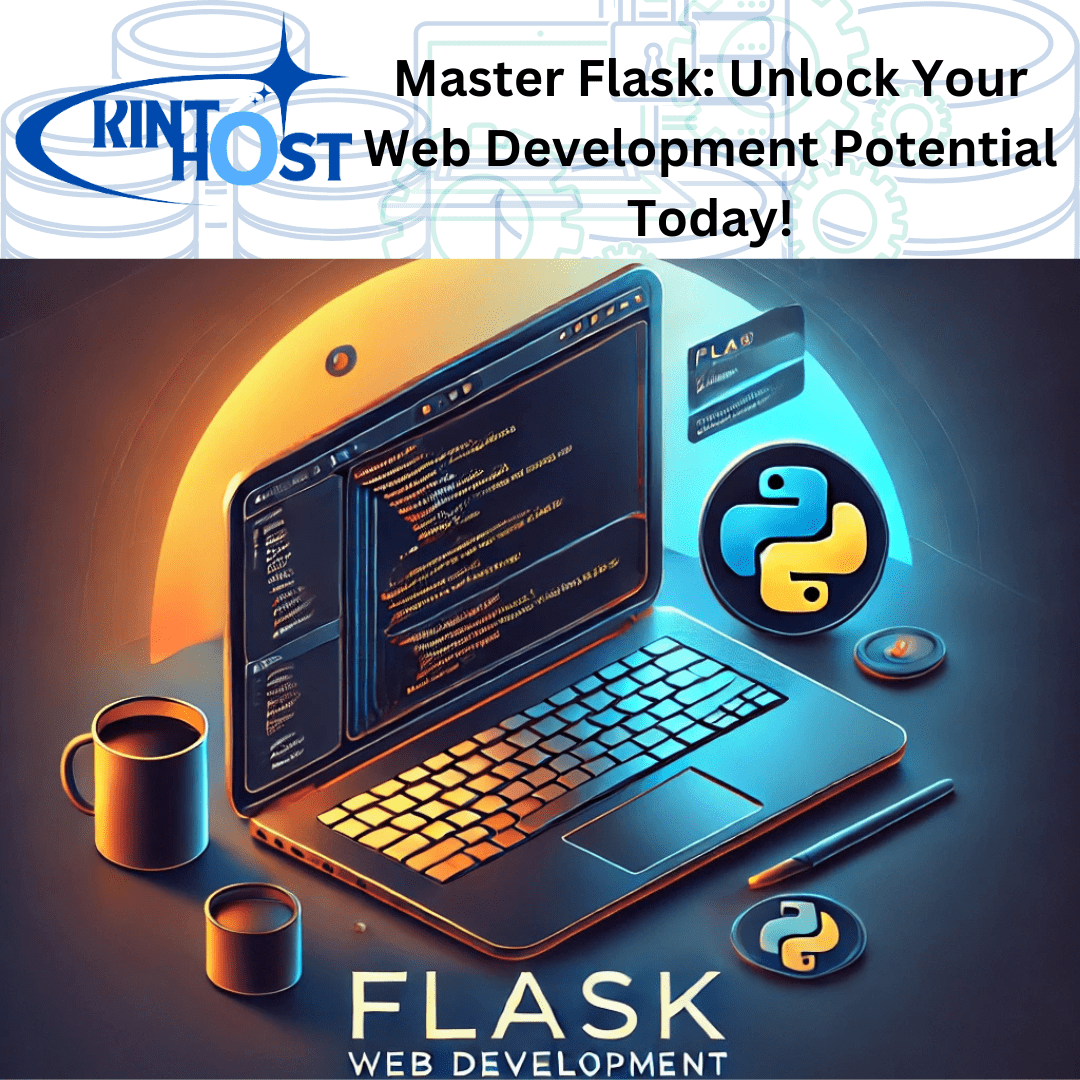
Flask web framework has emerged as a popular choice among developers for building dynamic web applications. Flask web framework Known for its simplicity, flexibility, and lightweight design, Flask empowers developers to craft scalable and robust applications. This guide delves into the core features, advantages, and best practices of using Flask web framework, ensuring an in-depth understanding of its potential.
What is Flask?
Flask web framework is a micro web framework written in Python. It was created by Armin Ronacher and first released in 2010. Despite being referred to as a “micro” framework, Flask provides ample functionality for building sophisticated web applications. The term “micro” signifies its minimalist approach—offering only the essential tools and features, while leaving the choice of additional libraries and extensions to the developer.
Key Features of Flask:
- Lightweight and Modular: Flask web framework is designed to be simple and unobtrusive, giving developers full control over their applications.
- Built-in Development Server: Flask web framework comes with a built-in server for testing and debugging purposes.
- Integrated Debugger: Flask includes a powerful debugger that simplifies the debugging process.
- Flexible Routing: The routing system allows developers to define URL patterns effortlessly.
- Support for Jinja2 Templates: Flask integrates seamlessly with Jinja2, a powerful templating engine.
- Extensibility: With its modular design, Flask can be extended using a wide range of plugins and extensions.
- Compatibility: Flask supports WSGI (Web Server Gateway Interface) applications and is compliant with Python 3.
Why Choose Flask?
Flask web framework is a preferred choice for various reasons, especially for developers seeking control and simplicity in their web applications.
1. Ease of Use:
Flask web framework straightforward syntax and minimal setup requirements make it beginner-friendly. Developers can quickly set up a project without the overhead of configuration.
2. Flexibility:
Unlike monolithic frameworks, Flask doesn’t enforce strict conventions. Developers have the freedom to choose libraries, database systems, and other components based on their project requirements.
3. Scalability:
Flask’s modular nature makes it suitable for both small applications and large-scale systems. As the application grows, additional features can be seamlessly integrated.
4. Active Community:
With a vibrant community, Flask web framework boasts extensive documentation, tutorials, and third-party resources, ensuring ample support for developers.
5. Versatility:
Flask web framework is well-suited for a variety of use cases, including:
- RESTful APIs
- Single-page applications (SPAs)
- Prototyping and Minimum Viable Products (MVPs)
Setting Up Flask
To begin with Flask, follow these steps:
1. Install Flask:
First, ensure you have Python installed. Then, use the following command to install Flask via pip:
pip install flask
2. Create a Simple Application:
Create a Python file (e.g., app.py
) and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Hello, Flask!'
if __name__ == '__main__':
app.run(debug=True)
Run the application using:
python app.py
Visit http://127.0.0.1:5000/
in your browser to see the output.
3. Directory Structure:
For larger projects, organize your files using a structured approach:
project_name/
app/
__init__.py
routes.py
templates/
static/
config.py
run.py
Flask’s Core Concepts
1. Routing:
Routing determines how URLs correspond to specific functions in your application. Flask’s @app.route
decorator simplifies this process.
@app.route('/about')
def about():
return 'About Page'
2. Templates:
Jinja2 templates enable dynamic content rendering. For instance: HTML Template (templates/home.html):
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>Welcome, {{ user }}!</h1>
</body>
</html>
Python Code:
from flask import render_template
@app.route('/home')
def home():
return render_template('home.html', title='Home Page', user='John Doe')
3. Forms and User Input:
Flask handles form data efficiently: HTML Form:
<form action="/submit" method="post">
<input type="text" name="name" placeholder="Enter your name">
<button type="submit">Submit</button>
</form>
Python Code:
from flask import request
@app.route('/submit', methods=['POST'])
def submit():
name = request.form['name']
return f'Hello, {name}!'
4. Database Integration:
Flask supports various database systems through extensions like SQLAlchemy:
from flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(80))
5. RESTful APIs:
Flask’s simplicity makes it ideal for building REST APIs:
from flask import jsonify
@app.route('/api/data')
def data():
return jsonify({'message': 'Hello, API'})
Best Practices for Using Flask
- Follow a Modular Structure: Organize your code into blueprints for better scalability and maintenance.
- Use Virtual Environments: Keep your project’s dependencies isolated with tools like
venv
orpipenv
. - Implement Security Measures: Protect your application with measures like input validation, CSRF tokens, and secure configurations.
- Leverage Extensions: Use Flask extensions such as Flask-RESTful, Flask-WTF, and Flask-Login to enhance functionality.
- Test Your Application: Write unit tests to ensure your application behaves as expected.
Conclusion
Flask web framework is a powerful and versatile framework that caters to a wide range of development needs. Its simplicity, combined with its extensibility, makes it an excellent choice for beginners and seasoned developers alike. By adhering to best practices and leveraging Flask’s rich ecosystem, you can build high-performing and secure web applications with ease.
Whether you’re prototyping a new idea or building a production-ready system, Flask’s flexibility ensures it can adapt to your project’s requirements. Start your Flask journey today and unlock the potential of this remarkable framework.
(FAQs):
- What is Flask used for in web development?
Flask is used to create web applications, RESTful APIs, and dynamic web services with a minimalist and flexible approach. - Is Flask better than Django?
Flask is better suited for lightweight, custom applications, while Django is ideal for larger, feature-rich projects. - Can Flask handle large applications?
Yes, Flask can scale to handle large applications when designed with a modular structure and best practices. - What makes Flask a “micro” framework?
Flask is considered a microframework because it provides essential tools without enforcing a specific architecture or including additional libraries by default. - How is Flask different from traditional frameworks?
Flask offers flexibility and simplicity, letting developers choose components and libraries as needed, unlike monolithic frameworks with built-in features. - What is Jinja2 in Flask?
Jinja2 is Flask’s templating engine, used to generate dynamic HTML content by embedding Python expressions in templates. - Can I use Flask with a database?
Yes, Flask web framework supports databases via extensions like SQLAlchemy, Flask-SQLAlchemy, and others. - Is Flask suitable for beginners?
Absolutely! Flask’s simplicity and beginner-friendly documentation make it an excellent choice for learning web development. - What are Flask extensions?
Flask extensions are add-ons that enhance Flask\u2019s functionality, such as Flask-RESTful for APIs, Flask-WTF for forms, and Flask-Login for authentication. - What are Flask\u2019s limitations?
Flask web framework does not provide built-in support for advanced features like authentication and user management, which must be implemented or added via extensions.